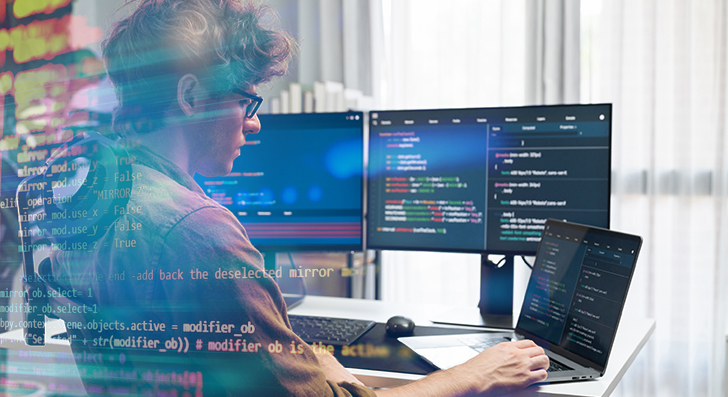
Scalability implies your software can cope with expansion—a lot more customers, extra facts, and even more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be element of your prepare from the beginning. A lot of applications fall short when they increase fast for the reason that the initial style can’t cope with the extra load. Like a developer, you might want to Feel early regarding how your system will behave under pressure.
Commence by building your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular design or microservices. These styles break your app into more compact, unbiased parts. Every single module or assistance can scale on its own with out impacting the whole technique.
Also, give thought to your database from day a single. Will it will need to take care of a million consumers or maybe a hundred? Select the appropriate form—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
One more significant issue is to avoid hardcoding assumptions. Don’t write code that only functions below existing situations. Think of what would come about if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that help scaling, like concept queues or occasion-pushed programs. These support your app manage a lot more requests without having acquiring overloaded.
Once you Construct with scalability in mind, you are not just getting ready for success—you're reducing future problems. A perfectly-prepared technique is simpler to maintain, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases can be a crucial Section of creating scalable applications. Not all databases are crafted the exact same, and using the wrong you can slow you down or even induce failures as your app grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to deal with more website traffic and information.
If the facts is more adaptable—like user action logs, products catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured knowledge and can scale horizontally a lot more conveniently.
Also, contemplate your examine and write designs. Are you presently carrying out numerous reads with fewer writes? Use caching and skim replicas. Are you dealing with a significant generate load? Explore databases which can deal with substantial generate throughput, or perhaps function-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need Superior scaling capabilities now, but deciding on a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Prevent unnecessary joins. Normalize or denormalize your information based on your accessibility designs. And generally observe databases general performance as you grow.
In short, the right database depends on your application’s composition, velocity wants, And the way you anticipate it to develop. Consider time to pick wisely—it’ll help you save a lot of trouble afterwards.
Enhance Code and Queries
Rapid code is key to scalability. As your application grows, each individual smaller hold off adds up. Badly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct successful logic from the start.
Begin by crafting cleanse, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy just one operates. Keep your features brief, concentrated, and simple to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual items down much more than the code by itself. Be certain Just about every question only asks for the info you really have to have. Stay away from SELECT *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Specifically throughout large tables.
In case you see exactly the same facts being requested time and again, use caching. Store the outcomes briefly applying resources like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional economical.
Remember to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are rapidly applications. Keep the code tight, your queries lean, and use caching when required. These measures support your application keep easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to deal with far more end users and a lot more website traffic. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across several servers. Rather than 1 server performing all the work, the load balancer routes buyers to unique servers based upon availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this very easy to here create.
Caching is about storing data quickly so it may be reused quickly. When people request the same facts yet again—like a product page or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick entry.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust usually. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but impressive resources. Jointly, they help your app take care of extra customers, keep speedy, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your app develop simply. That’s where by cloud platforms and containers come in. They give you versatility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential capability. When targeted traffic boosts, you may insert more resources with just a few clicks or automatically utilizing auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You may center on making your application as opposed to handling infrastructure.
Containers are An additional crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This makes it easy to maneuver your app in between environments, from a notebook for the cloud, with out surprises. Docker is the preferred tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy simply, and recover speedily when issues come about. If you would like your application to mature without having restrictions, begin working with these equipment early. They help you save time, minimize possibility, and assist you to keep centered on building, not repairing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make superior conclusions as your app grows. It’s a important Section of making scalable units.
Begin by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Regulate how long it requires for consumers to load webpages, how often mistakes take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a limit or a company goes down, you'll want to get notified straight away. This can help you take care of challenges rapid, generally ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. In the event you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic injury.
As your app grows, website traffic and info improve. Without the need of monitoring, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking aids you keep your app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it really works properly, even stressed.
Last Views
Scalability isn’t just for major businesses. Even smaller apps have to have a powerful Basis. By designing meticulously, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create good.